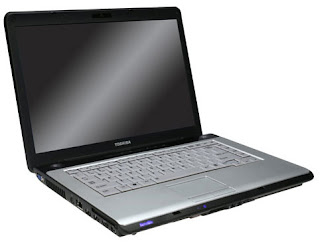
Hopefully i can do this here...
It’s finally here! Down to earth, simple to read, simple to understand tutorial on how to install Mac OS X on Toshiba Satellite A205-S5804 model. If you read my previous article on this subject, you know that it is possible mainly because of Apple’s move to Intel chips. (Wait, did I write about it before?) Anyway, I did a lot of research and have come to believe that Toshiba Satellite is one of the easiest laptops to get Mac OS X working. And by working I mean full video support, sound, Wi-Fi, Ethernet, usb ports, sound adjuster, battery meter, restart and shutdown. Plus, you can run it all on vanilla kernel (original apple kernel).
Toshiba laptops are relatively cheap. Besides, if you want to spend lots of money just to create ‘hackintosh’, I suggest you buying a real Mac instead. Why? Simply because OS X was designed to run on Apple hardware. You won’t have any issues or problems if you run major software update straight from the Apple Software Update. But if you do the same thing on the Toshiba for example, you might get ‘spinning circle of death’ (ha ha, you never heard of the term 'spinning cirlce of death?; you heard 'blue screen of death', havent you?) after reboot. Some can argue that it’s not a big deal if you use modified updates from hackers (good ones) however, you must be willing to spend some time getting your system up to date. (Honestly, it’s not a big deal.)
This tutorial is for educational purposes only. You, of course, assume all responsibility for choosing to follow this tutorial. Also, OS X EULA states that you can run Leopard on Apple labeled hardware only.
Let’s get to work. As I said I use Toshiba Satellite A205-5804. A little of specifications won’t hurt. So here you go, download it from
here.
You will need a modified OS X installation DVD with modified boot loader. It will allow you to load, and install OS X with no problems. This is the result of BIOS not being able to recognize Retail OS X DVD as bootable. For this install, I use ‘leo4allv3’.
Here is what you need:
• Leo4allv3 (Google it)
• Drivers (Kext) –
here.
• Kalyway 10.5.3 Combo Update with Kernel package (dont ask me where to find it, Google it)
1. Burn your leo4allv3 ISO to a DVD
2. Changing BIOS Settings:
• In BIOS settings, make sure DVD drive is selected as the first booting device. This is required in order to install OS X. No need to change anything else.
3. Booting/Installing leo4allv3:
• Place your leo4allv3 DVD into DVD drive and turn on/restart your laptop.
• At the Darwin prompt, you will read this: “Press any key to start from CD-Rom, or press F8 to enter start up options…” You need to press F8 and type ‘-v’ (without ‘) and press ‘Enter’ to load OS X in verbose mode. After few minutes you should see a window with a choice of different languages. Choose your own and click on ‘next’ button.
• After you see a ‘Welcome Screen’, make sure you don’t click on ‘Continue’ button yet. You need to format your hard drive first. Go to ‘Utilities → Disc utility’. (You should be able to see ‘Utilities’ option on the top bar.)
• Select your hard drive at the top left corner and click on ‘Erase’ tab (located in the middle of the window).
• Choose ‘Mac OS Extended (Journaled)’ from the drop down menu of ‘Volume Format’ and click on ‘Erase’ button. Confirm the erase command.
• Click on ‘Partition’ tab and in the ‘Volume Scheme’ drop down menu choose – 1 Partition. In the Name field type ‘OSX’. Leave the format option to ‘Mac OS Extended (Journaled).
• Now, click on ‘Options’ button at the bottom left corner and choose ‘Master Boot Record’ (MBR), click on OK and hit ‘Apply’ at the main ‘Disk Utility’ window. Confirm the creation of the ‘OSX’ partition. Wait few moments as ‘Disk Utility’ works its way through.
• Close ‘Disk Utility’ by clicking on the red dot at the top of the ‘Disk Utility’ window. You are back to the ‘Welcome’ screen.
• Click on ‘Continue’ button and hit ‘Agree’.
• Select destination hard drive – OSX and click on ‘Continue’.
• Click on ‘Customize’ button just before you click on ‘Install’ at 'Install Summary' window.
• Choose these packages: Under Audio, choose Azalia Audio; under Chipset, don’t select anything; under Kernel option, choose 9.2.0 sleep; under (SMBIOS), choose Apple SMBIOS 667; under Video choose GMAX3100; under Applications, choose all; select Remove Firewire; select Power Management for laptops; select About This Mac; Click on ‘Done’ button.
• After you get back to ‘Install Summary’, click on ‘Install’ (you can skip checking install DVD by hitting ‘skip’ button).
• Installing - wait. At some point it will look like the installation process got stock, its normal. Just wait.
• After you see ‘Install Succeeded’ window, click on ‘Restart’ button.
4. Fixing minor bugs:
Note: Don’t let OS X boot normally, it will most likely get stuck on a cycled reboot at ‘Transfer Your Information Window’ later, during account setup. Instead Press F8 at Darwin boot prompt and type: -v –s (hit enter);
• After you see ‘root#’, type ‘/sbin/fsck –fy’ and hit ‘Enter’ Type /sbin/mount -uw / and hit ‘Enter’ again. (it’s going to take a little time to run script, wait)
• Type ‘touch /var/db/.AppleSetupDone’ and hit Enter;
• You will need to set up password for root, type: passwd (yes, passwd) and hit enter; choose your password.
Note: when you log in for the first time, your user name will be: root and password is the one you set up.
• Now, after you log in, set up your personal account. (It’s not recommended to use ‘Root Account’) Under Settings User Account, you will have an option of creating an account, do that.
• As you see, you DVD drive don’t show up, you will have to fix it. There is a driver in ‘Driver Folder’ you downloaded earlier called ‘IOATAFamily.kext_V1.1’, open it and install it with KextHelper. (Google it)
• If you want to change default Verbose Loading mode to Regular, do this: go to /Library/Preferences/SystemConfiguration/com.apple.Boot.plist. Open it with BBEdit delete ‘-v’ from the list and it will boot normally.
• To fix Wi-Fi, install rtl8187b drive from the Drivers folder. Restart if needed. Go to 'system/library/extensions folder and find rtl8187.kext' Open it with TextMate and find this line:
33161 and change numbers to 33175. Restart your computer. (Don’t freak out if you don’t see your Wi-Fi connection yet, you will have to install 10.5.3 combo update to make it work as it should!)
5. Updating to 10.5.3:
• Open your folder with Kalyway 10.5.3 Combo Update, and double click on Kalyway UpdCombo10.5.3.pkg to initiate install. Confirm it by entering your password. Note: Do not click on ‘Restart’ after successful install, as it most likely give you lots of problems on boot. Rather leave the window open and navigate to ‘Kalyway10.5.3 Kernel’ (it should have been in the same folder with kalyway combo update). Double click on it to open the installer, and install it WITHOUT selecting any kernel versions. Close the window, get back to 10.5.3 Combo Update window and click on ‘Restart’. (You should be able to restart successfully)
• Power management will not work after 10.5.3 update. To make it work, install Power Management 10.5.3 fix from drivers’ folder.
6. Updating to 10.5.4:
• Navigate to Apple Software Update from your desktop by clicking on ‘Apple’ logo at the top left corner and choosing ‘Software Update’. It will check if your system is up to date, which of course not, so it will show you that 10.5.4 is available. Install it and reboot. Now you should have completely working ‘Mac’ running on latest OS X.
Congratulations on your 100% working ToshiMac!!! If you found this article interesting, please leave a comment. By the way comments are always welcome, so please take 30 seconds of your time to write one. If you want to share your experience, please do so by writing below.